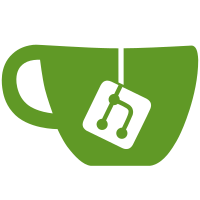
This commit contains a new utility that helps to automate a version bump for sandbox. You can run this command to get the vibe of what it does. ``` $ go run cmd/bump-version/main.go -help ``` In order to try it out you can run this command form sandbox root. By default it won't overwrite anything. It will print to stdout a new version of the current_versions.sh file where all the images are calculate cloning the various repositories ``` $ go run cmd/bump-version/main.go ``` If you want to overwrite the current_versions file you can use the flag `-overwrite`. More will come but for now, that's the PoC. Ideally this can be hooked to CI/CD and run periodically, opening a PR that can be evaluated and merged. Signed-off-by: Gianluca Arbezzano <gianarb92@gmail.com>
49 lines
979 B
Go
49 lines
979 B
Go
package envfile
|
|
|
|
import (
|
|
"fmt"
|
|
"sort"
|
|
"strings"
|
|
|
|
"github.com/joho/godotenv"
|
|
)
|
|
|
|
type EnvFile map[string]string
|
|
|
|
func ReadEnvFile(f string) (EnvFile, error) {
|
|
myEnv, err := godotenv.Read(f)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return myEnv, nil
|
|
}
|
|
|
|
// Copied and modified from https://github.com/joho/godotenv
|
|
|
|
const doubleQuoteSpecialChars = "\\\n\r\"!$`"
|
|
|
|
func Marshal(envMap map[string]string) (string, error) {
|
|
lines := make([]string, 0, len(envMap))
|
|
for k, v := range envMap {
|
|
lines = append(lines, fmt.Sprintf(`export %s="%s"`, k, doubleQuoteEscape(v)))
|
|
}
|
|
sort.Strings(lines)
|
|
return strings.Join(lines, "\n"), nil
|
|
}
|
|
|
|
func doubleQuoteEscape(line string) string {
|
|
for _, c := range doubleQuoteSpecialChars {
|
|
toReplace := "\\" + string(c)
|
|
if c == '\n' {
|
|
toReplace = `\n`
|
|
}
|
|
if c == '\r' {
|
|
toReplace = `\r`
|
|
}
|
|
line = strings.Replace(line, string(c), toReplace, -1)
|
|
}
|
|
return line
|
|
}
|
|
|
|
// End of the part copied from https://github.com/joho/godotenv
|