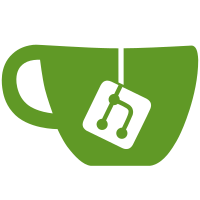
This required a weird hack because some of the Fosite tests (or a transitive dependency of them) depends on a newer version of gRPC that's incompatible with the Kubernetes runtime version we use. It wasn't as simple as just replacing the gRPC module with an older version, because in the latest versions of gRPC, they split out the "examples" packages into their own module. This new module name doesn't exist at the old version. Ultimately, the workaround was to make a fake "examples" module locally. This module can be empty because we never actually depend on that code (it's only used in transitive dependency tests). Signed-off-by: Matt Moyer <moyerm@vmware.com>
90 lines
2.0 KiB
Bash
Executable File
90 lines
2.0 KiB
Bash
Executable File
#!/usr/bin/env bash
|
|
|
|
# Copyright 2020-2021 the Pinniped contributors. All Rights Reserved.
|
|
# SPDX-License-Identifier: Apache-2.0
|
|
|
|
set -euo pipefail
|
|
|
|
ROOT="$(cd "$(dirname "${BASH_SOURCE[0]}")/.." && pwd)"
|
|
|
|
function tidy_cmd() {
|
|
echo 'go mod tidy -v'
|
|
}
|
|
|
|
function lint_cmd() {
|
|
echo "golangci-lint run --modules-download-mode=readonly --timeout=10m"
|
|
}
|
|
|
|
function test_cmd() {
|
|
if [ -x "$(command -v gotest)" ]; then
|
|
cmd='gotest'
|
|
else
|
|
cmd='go test'
|
|
fi
|
|
echo "${cmd} -count 1 -race ./..."
|
|
}
|
|
|
|
function unittest_cmd() {
|
|
if [ -x "$(command -v gotest)" ]; then
|
|
cmd='gotest'
|
|
else
|
|
cmd='go test'
|
|
fi
|
|
echo "${cmd} -short -race ./..."
|
|
}
|
|
|
|
function with_modules() {
|
|
local cmd_function="${1}"
|
|
cmd="$(${cmd_function})"
|
|
|
|
# start the cache mutation detector by default so that cache mutators will be found
|
|
local kube_cache_mutation_detector="${KUBE_CACHE_MUTATION_DETECTOR:-true}"
|
|
|
|
# panic the server on watch decode errors since they are considered coder mistakes
|
|
local kube_panic_watch_decode_error="${KUBE_PANIC_WATCH_DECODE_ERROR:-true}"
|
|
|
|
env_vars="KUBE_CACHE_MUTATION_DETECTOR=${kube_cache_mutation_detector} KUBE_PANIC_WATCH_DECODE_ERROR=${kube_panic_watch_decode_error}"
|
|
|
|
pushd "${ROOT}" >/dev/null
|
|
for mod_file in $(find . -maxdepth 4 -not -path "./generated/*" -not -path "./hack/*" -name go.mod | sort); do
|
|
mod_dir="$(dirname "${mod_file}")"
|
|
(
|
|
echo "=> "
|
|
echo " cd ${mod_dir} && ${env_vars} ${cmd}"
|
|
cd "${mod_dir}" && env ${env_vars} ${cmd}
|
|
)
|
|
done
|
|
popd >/dev/null
|
|
}
|
|
|
|
function usage() {
|
|
echo "Error: <task> must be specified"
|
|
echo " module.sh <task> [tidy, lint, test, unittest]"
|
|
exit 1
|
|
}
|
|
|
|
function main() {
|
|
case "${1:-invalid}" in
|
|
'tidy')
|
|
with_modules 'tidy_cmd'
|
|
;;
|
|
'lint' | 'linter' | 'linters')
|
|
with_modules 'lint_cmd'
|
|
;;
|
|
'test' | 'tests')
|
|
with_modules 'test_cmd'
|
|
;;
|
|
'unittest' | 'unittests' | 'units' | 'unit')
|
|
with_modules 'unittest_cmd'
|
|
;;
|
|
*)
|
|
usage
|
|
;;
|
|
esac
|
|
|
|
echo "=> "
|
|
echo " \"module.sh $1\" Finished successfully."
|
|
}
|
|
|
|
main "$@"
|