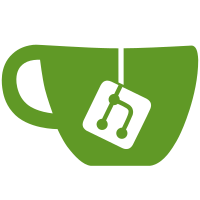
This is a partial revert of 288d9c999e
. For some reason it didn't occur to me
that we could do it this way earlier. Whoops.
This also contains a middleware update: mutation funcs can return an error now
and short-circuit the rest of the request/response flow. The idea here is that
if someone is configuring their kubeclient to use middleware, they are agreeing
to a narrow-er client contract by doing so (e.g., their TokenCredentialRequest's
must have an Spec.Authenticator.APIGroup set).
I also updated some internal/groupsuffix tests to be more realistic.
Signed-off-by: Andrew Keesler <akeesler@vmware.com>
76 lines
1.8 KiB
Go
76 lines
1.8 KiB
Go
// Copyright 2021 the Pinniped contributors. All Rights Reserved.
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package kubeclient
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/require"
|
|
corev1 "k8s.io/api/core/v1"
|
|
metav1 "k8s.io/apimachinery/pkg/apis/meta/v1"
|
|
"k8s.io/apimachinery/pkg/runtime/schema"
|
|
)
|
|
|
|
func Test_request_mutate(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
reqFuncs []func(Object) error
|
|
obj Object
|
|
want *mutationResult
|
|
wantObj Object
|
|
wantErr string
|
|
}{
|
|
{
|
|
name: "mutate config map data",
|
|
reqFuncs: []func(Object) error{
|
|
func(obj Object) error {
|
|
cm := obj.(*corev1.ConfigMap)
|
|
cm.Data = map[string]string{"new": "stuff"}
|
|
return nil
|
|
},
|
|
},
|
|
obj: &corev1.ConfigMap{
|
|
TypeMeta: metav1.TypeMeta{APIVersion: "v1", Kind: "ConfigMap"},
|
|
Data: map[string]string{"old": "things"},
|
|
BinaryData: map[string][]byte{"weee": nil},
|
|
},
|
|
want: &mutationResult{
|
|
origGVK: schema.GroupVersionKind{Group: "", Version: "v1", Kind: "ConfigMap"},
|
|
newGVK: schema.GroupVersionKind{Group: "", Version: "v1", Kind: "ConfigMap"},
|
|
gvkChanged: false,
|
|
mutated: true,
|
|
},
|
|
wantObj: &corev1.ConfigMap{
|
|
TypeMeta: metav1.TypeMeta{APIVersion: "v1", Kind: "ConfigMap"},
|
|
Data: map[string]string{"new": "stuff"},
|
|
BinaryData: map[string][]byte{"weee": nil},
|
|
},
|
|
wantErr: "",
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
tt := tt
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
r := &request{reqFuncs: tt.reqFuncs}
|
|
orig := tt.obj.DeepCopyObject()
|
|
|
|
got, err := r.mutateRequest(tt.obj)
|
|
|
|
if len(tt.wantErr) > 0 {
|
|
require.EqualError(t, err, tt.wantErr)
|
|
} else {
|
|
require.NoError(t, err)
|
|
}
|
|
|
|
require.Equal(t, tt.want, got)
|
|
|
|
if tt.wantObj != nil {
|
|
require.Equal(t, tt.wantObj, tt.obj)
|
|
} else {
|
|
require.Equal(t, orig, tt.obj)
|
|
}
|
|
})
|
|
}
|
|
}
|