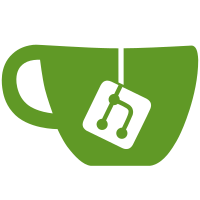
Before this change, we used the `fosite.DefaultOpenIDConnectClient{}` struct, which implements the `fosite.Client` and `fosite.OpenIDConnectClient` interfaces. For a future change, we also need to implement some additional optional interfaces, so we can no longer use the provided default types. Instead, we now use a custom `clientregistry.Client{}` struct, which implements all the requisite interfaces and can be extended to handle the new functionality (in a future change). There is also a new `clientregistry.StaticRegistry{}` struct, which implements the `fosite.ClientManager` and looks up our single static client. We could potentially extend this in the future with a registry backed by Kubernetes API, for example. This should be 100% refactor, with no user-observable change. Signed-off-by: Matt Moyer <moyerm@vmware.com>
37 lines
1.1 KiB
Go
37 lines
1.1 KiB
Go
// Copyright 2020-2021 the Pinniped contributors. All Rights Reserved.
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package fositestorage
|
|
|
|
import (
|
|
"github.com/ory/fosite"
|
|
"github.com/ory/fosite/handler/openid"
|
|
|
|
"go.pinniped.dev/internal/constable"
|
|
"go.pinniped.dev/internal/oidc/clientregistry"
|
|
)
|
|
|
|
const (
|
|
ErrInvalidRequestType = constable.Error("requester must be of type fosite.Request")
|
|
ErrInvalidClientType = constable.Error("requester's client must be of type clientregistry.Client")
|
|
ErrInvalidSessionType = constable.Error("requester's session must be of type openid.DefaultSession")
|
|
StorageRequestIDLabelName = "storage.pinniped.dev/request-id" //nolint:gosec // this is not a credential
|
|
)
|
|
|
|
func ValidateAndExtractAuthorizeRequest(requester fosite.Requester) (*fosite.Request, error) {
|
|
request, ok1 := requester.(*fosite.Request)
|
|
if !ok1 {
|
|
return nil, ErrInvalidRequestType
|
|
}
|
|
_, ok2 := request.Client.(*clientregistry.Client)
|
|
if !ok2 {
|
|
return nil, ErrInvalidClientType
|
|
}
|
|
_, ok3 := request.Session.(*openid.DefaultSession)
|
|
if !ok3 {
|
|
return nil, ErrInvalidSessionType
|
|
}
|
|
|
|
return request, nil
|
|
}
|