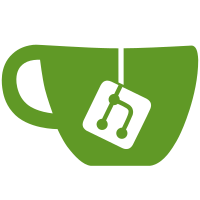
This is a partial revert of 288d9c999e
. For some reason it didn't occur to me
that we could do it this way earlier. Whoops.
This also contains a middleware update: mutation funcs can return an error now
and short-circuit the rest of the request/response flow. The idea here is that
if someone is configuring their kubeclient to use middleware, they are agreeing
to a narrow-er client contract by doing so (e.g., their TokenCredentialRequest's
must have an Spec.Authenticator.APIGroup set).
I also updated some internal/groupsuffix tests to be more realistic.
Signed-off-by: Andrew Keesler <akeesler@vmware.com>
55 lines
1.2 KiB
Go
55 lines
1.2 KiB
Go
// Copyright 2021 the Pinniped contributors. All Rights Reserved.
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package kubeclient
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func Test_verb(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
f func() string
|
|
want string
|
|
}{
|
|
{
|
|
name: "error: string format",
|
|
f: func() string {
|
|
return fmt.Errorf("%s", VerbGet).Error()
|
|
},
|
|
want: "get",
|
|
},
|
|
{
|
|
name: "error: value format",
|
|
f: func() string {
|
|
return fmt.Errorf("%v", VerbUpdate).Error()
|
|
},
|
|
want: "update",
|
|
},
|
|
{
|
|
name: "error: go value format",
|
|
f: func() string {
|
|
return fmt.Errorf("%#v", VerbDelete).Error()
|
|
},
|
|
want: `"delete"`,
|
|
},
|
|
{
|
|
name: "error: go value format in middelware request",
|
|
f: func() string {
|
|
return fmt.Errorf("%#v", request{verb: VerbPatch}).Error()
|
|
},
|
|
want: `kubeclient.request{verb:"patch", namespace:"", resource:schema.GroupVersionResource{Group:"", Version:"", Resource:""}, reqFuncs:[]func(kubeclient.Object) error(nil), respFuncs:[]func(kubeclient.Object) error(nil), subresource:""}`,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
tt := tt
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
require.Equal(t, tt.want, tt.f())
|
|
})
|
|
}
|
|
}
|