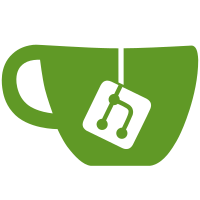
- Refactors the existing cert generation code into controllers which read and write a Secret containing the certs - Does not add any new functionality yet, e.g. no new handling for cert expiration, and no leader election to allow for multiple servers running simultaneously - This commit also doesn't add new tests for the cert generation code, but it should be more unit testable now as controllers
70 lines
2.2 KiB
Go
70 lines
2.2 KiB
Go
/*
|
|
Copyright 2020 VMware, Inc.
|
|
SPDX-License-Identifier: Apache-2.0
|
|
*/
|
|
|
|
package apicerts
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
k8serrors "k8s.io/apimachinery/pkg/api/errors"
|
|
corev1informers "k8s.io/client-go/informers/core/v1"
|
|
"k8s.io/klog/v2"
|
|
|
|
"github.com/suzerain-io/controller-go"
|
|
placeholdernamecontroller "github.com/suzerain-io/placeholder-name/internal/controller"
|
|
"github.com/suzerain-io/placeholder-name/internal/provider"
|
|
)
|
|
|
|
type certsObserverController struct {
|
|
namespace string
|
|
dynamicCertProvider *provider.DynamicTLSServingCertProvider
|
|
secretInformer corev1informers.SecretInformer
|
|
}
|
|
|
|
func NewCertsObserverController(
|
|
namespace string,
|
|
dynamicCertProvider *provider.DynamicTLSServingCertProvider,
|
|
secretInformer corev1informers.SecretInformer,
|
|
withInformer placeholdernamecontroller.WithInformerOptionFunc,
|
|
) controller.Controller {
|
|
return controller.New(
|
|
controller.Config{
|
|
Name: "certs-observer-controller",
|
|
Syncer: &certsObserverController{
|
|
namespace: namespace,
|
|
dynamicCertProvider: dynamicCertProvider,
|
|
secretInformer: secretInformer,
|
|
},
|
|
},
|
|
withInformer(
|
|
secretInformer,
|
|
placeholdernamecontroller.NameAndNamespaceExactMatchFilterFactory(certsSecretName, namespace),
|
|
controller.InformerOption{},
|
|
),
|
|
)
|
|
}
|
|
|
|
func (c *certsObserverController) Sync(_ controller.Context) error {
|
|
// Try to get the secret from the informer cache.
|
|
certSecret, err := c.secretInformer.Lister().Secrets(c.namespace).Get(certsSecretName)
|
|
notFound := k8serrors.IsNotFound(err)
|
|
if err != nil && !notFound {
|
|
return fmt.Errorf("failed to get %s/%s secret: %w", c.namespace, certsSecretName, err)
|
|
}
|
|
if notFound {
|
|
klog.Info("certsObserverController Sync() found that the secret does not exist yet or was deleted")
|
|
// The secret does not exist yet or was deleted.
|
|
c.dynamicCertProvider.CertPEM = nil
|
|
c.dynamicCertProvider.KeyPEM = nil
|
|
return nil
|
|
}
|
|
|
|
// Mutate the in-memory cert provider to update with the latest cert values.
|
|
c.dynamicCertProvider.CertPEM = certSecret.Data[tlsCertificateChainSecretKey]
|
|
c.dynamicCertProvider.KeyPEM = certSecret.Data[tlsPrivateKeySecretKey]
|
|
klog.Info("certsObserverController Sync updated certs in the dynamic cert provider")
|
|
return nil
|
|
}
|