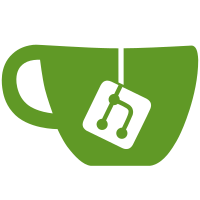
We are getting these weird flakes in CI where the kube client that we create with these helper functions doesn't work against the kube API. The kube API tells us that we are unauthorized (401). Seems like something is wrong with the keypair itself, but when I create a one-off kubeconfig with the keypair, I get 200s from the API. Hmmm...I wonder what CI will think of this change? I also tried to align some naming in this package. Signed-off-by: Andrew Keesler <akeesler@vmware.com>
148 lines
4.3 KiB
Go
148 lines
4.3 KiB
Go
/*
|
|
Copyright 2020 VMware, Inc.
|
|
SPDX-License-Identifier: Apache-2.0
|
|
*/
|
|
|
|
package library
|
|
|
|
import (
|
|
"io/ioutil"
|
|
"os"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/require"
|
|
"k8s.io/client-go/kubernetes"
|
|
"k8s.io/client-go/rest"
|
|
"k8s.io/client-go/tools/clientcmd"
|
|
clientcmdapi "k8s.io/client-go/tools/clientcmd/api"
|
|
aggregatorclient "k8s.io/kube-aggregator/pkg/client/clientset_generated/clientset"
|
|
|
|
pinnipedclientset "github.com/suzerain-io/pinniped/kubernetes/1.19/client-go/clientset/versioned"
|
|
)
|
|
|
|
func NewClientConfig(t *testing.T) *rest.Config {
|
|
t.Helper()
|
|
|
|
return newClientConfigWithOverrides(t, &clientcmd.ConfigOverrides{})
|
|
}
|
|
|
|
func NewClientset(t *testing.T) kubernetes.Interface {
|
|
t.Helper()
|
|
|
|
return newClientsetWithConfig(t, NewClientConfig(t))
|
|
}
|
|
|
|
func NewClientsetWithCertAndKey(t *testing.T, clientCertificateData, clientKeyData string) kubernetes.Interface {
|
|
t.Helper()
|
|
|
|
return newClientsetWithConfig(t, newClientConfigWithCertAndKey(t, clientCertificateData, clientKeyData))
|
|
}
|
|
|
|
func NewPinnipedClientset(t *testing.T) pinnipedclientset.Interface {
|
|
t.Helper()
|
|
|
|
return pinnipedclientset.NewForConfigOrDie(NewClientConfig(t))
|
|
}
|
|
|
|
func NewAnonymousPinnipedClientset(t *testing.T) pinnipedclientset.Interface {
|
|
t.Helper()
|
|
|
|
return pinnipedclientset.NewForConfigOrDie(newAnonymousClientConfig(t))
|
|
}
|
|
|
|
func NewAggregatedClientset(t *testing.T) aggregatorclient.Interface {
|
|
t.Helper()
|
|
|
|
return aggregatorclient.NewForConfigOrDie(NewClientConfig(t))
|
|
}
|
|
|
|
func newClientConfigWithOverrides(t *testing.T, overrides *clientcmd.ConfigOverrides) *rest.Config {
|
|
t.Helper()
|
|
|
|
loader := clientcmd.NewDefaultClientConfigLoadingRules()
|
|
clientConfig := clientcmd.NewNonInteractiveDeferredLoadingClientConfig(loader, overrides)
|
|
config, err := clientConfig.ClientConfig()
|
|
require.NoError(t, err)
|
|
return config
|
|
}
|
|
|
|
func newClientsetWithConfig(t *testing.T, config *rest.Config) kubernetes.Interface {
|
|
t.Helper()
|
|
|
|
result, err := kubernetes.NewForConfig(config)
|
|
require.NoError(t, err, "unexpected failure from kubernetes.NewForConfig()")
|
|
return result
|
|
}
|
|
|
|
// Returns a rest.Config without any user authentication info.
|
|
// Ensures that we are not accidentally picking up any authentication info from the kube config file.
|
|
// E.g. If your kube config were pointing at an Azure cluster, it would have both certs and a token,
|
|
// and we don't want our tests to accidentally pick up that token.
|
|
func newAnonymousClientConfig(t *testing.T) *rest.Config {
|
|
t.Helper()
|
|
|
|
realConfig := NewClientConfig(t)
|
|
|
|
out, err := ioutil.TempFile("", "pinniped-anonymous-kubeconfig-test-*")
|
|
require.NoError(t, err)
|
|
defer os.Remove(out.Name())
|
|
|
|
anonConfig := clientcmdapi.NewConfig()
|
|
anonConfig.Clusters["anonymous-cluster"] = &clientcmdapi.Cluster{
|
|
Server: realConfig.Host,
|
|
CertificateAuthorityData: realConfig.CAData,
|
|
}
|
|
anonConfig.Contexts["anonymous"] = &clientcmdapi.Context{
|
|
Cluster: "anonymous-cluster",
|
|
}
|
|
anonConfig.CurrentContext = "anonymous"
|
|
|
|
data, err := clientcmd.Write(*anonConfig)
|
|
require.NoError(t, err)
|
|
|
|
_, err = out.Write(data)
|
|
require.NoError(t, err)
|
|
|
|
restConfig, err := clientcmd.BuildConfigFromFlags("", out.Name())
|
|
require.NoError(t, err)
|
|
|
|
return restConfig
|
|
}
|
|
|
|
// Starting with an anonymous client config, add a cert and key to use for authentication in the API server.
|
|
func newClientConfigWithCertAndKey(t *testing.T, clientCertificateData, clientKeyData string) *rest.Config {
|
|
t.Helper()
|
|
|
|
realConfig := NewClientConfig(t)
|
|
|
|
out, err := ioutil.TempFile("", "pinniped-cert-and-key-kubeconfig-test-*")
|
|
require.NoError(t, err)
|
|
defer os.Remove(out.Name())
|
|
|
|
certAndKeyConfig := clientcmdapi.NewConfig()
|
|
certAndKeyConfig.Clusters["cert-and-key-cluster"] = &clientcmdapi.Cluster{
|
|
Server: realConfig.Host,
|
|
CertificateAuthorityData: realConfig.CAData,
|
|
}
|
|
certAndKeyConfig.AuthInfos["cert-and-key-auth-info"] = &clientcmdapi.AuthInfo{
|
|
ClientCertificateData: []byte(clientCertificateData),
|
|
ClientKeyData: []byte(clientKeyData),
|
|
}
|
|
certAndKeyConfig.Contexts["cert-and-key"] = &clientcmdapi.Context{
|
|
Cluster: "cert-and-key-cluster",
|
|
AuthInfo: "cert-and-key-auth-info",
|
|
}
|
|
certAndKeyConfig.CurrentContext = "cert-and-key"
|
|
|
|
data, err := clientcmd.Write(*certAndKeyConfig)
|
|
require.NoError(t, err)
|
|
|
|
_, err = out.Write(data)
|
|
require.NoError(t, err)
|
|
|
|
restConfig, err := clientcmd.BuildConfigFromFlags("", out.Name())
|
|
require.NoError(t, err)
|
|
|
|
return restConfig
|
|
}
|