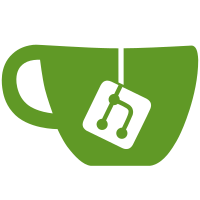
This change updates the TLS config used by all pinniped components. There are no configuration knobs associated with this change. Thus this change tightens our static defaults. There are four TLS config levels: 1. Secure (TLS 1.3 only) 2. Default (TLS 1.2+ best ciphers that are well supported) 3. Default LDAP (TLS 1.2+ with less good ciphers) 4. Legacy (currently unused, TLS 1.2+ with all non-broken ciphers) Highlights per component: 1. pinniped CLI - uses "secure" config against KAS - uses "default" for all other connections 2. concierge - uses "secure" config as an aggregated API server - uses "default" config as a impersonation proxy API server - uses "secure" config against KAS - uses "default" config for JWT authenticater (mostly, see code) - no changes to webhook authenticater (see code) 3. supervisor - uses "default" config as a server - uses "secure" config against KAS - uses "default" config against OIDC IDPs - uses "default LDAP" config against LDAP IDPs Signed-off-by: Monis Khan <mok@vmware.com>
181 lines
6.9 KiB
Go
181 lines
6.9 KiB
Go
// Copyright 2020-2021 the Pinniped contributors. All Rights Reserved.
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
// Package plog implements a thin layer over klog to help enforce pinniped's logging convention.
|
|
// Logs are always structured as a constant message with key and value pairs of related metadata.
|
|
//
|
|
// The logging levels in order of increasing verbosity are:
|
|
// error, warning, info, debug, trace and all.
|
|
//
|
|
// error and warning logs are always emitted (there is no way for the end user to disable them),
|
|
// and thus should be used sparingly. Ideally, logs at these levels should be actionable.
|
|
//
|
|
// info should be reserved for "nice to know" information. It should be possible to run a production
|
|
// pinniped server at the info log level with no performance degradation due to high log volume.
|
|
// debug should be used for information targeted at developers and to aid in support cases. Care must
|
|
// be taken at this level to not leak any secrets into the log stream. That is, even though debug may
|
|
// cause performance issues in production, it must not cause security issues in production.
|
|
//
|
|
// trace should be used to log information related to timing (i.e. the time it took a controller to sync).
|
|
// Just like debug, trace should not leak secrets into the log stream. trace will likely leak information
|
|
// about the current state of the process, but that, along with performance degradation, is expected.
|
|
//
|
|
// all is reserved for the most verbose and security sensitive information. At this level, full request
|
|
// metadata such as headers and parameters along with the body may be logged. This level is completely
|
|
// unfit for production use both from a performance and security standpoint. Using it is generally an
|
|
// act of desperation to determine why the system is broken.
|
|
package plog
|
|
|
|
import "k8s.io/klog/v2"
|
|
|
|
const errorKey = "error"
|
|
|
|
type Logger interface {
|
|
Error(msg string, err error, keysAndValues ...interface{})
|
|
Warning(msg string, keysAndValues ...interface{})
|
|
WarningErr(msg string, err error, keysAndValues ...interface{})
|
|
Info(msg string, keysAndValues ...interface{})
|
|
InfoErr(msg string, err error, keysAndValues ...interface{})
|
|
Debug(msg string, keysAndValues ...interface{})
|
|
DebugErr(msg string, err error, keysAndValues ...interface{})
|
|
Trace(msg string, keysAndValues ...interface{})
|
|
TraceErr(msg string, err error, keysAndValues ...interface{})
|
|
All(msg string, keysAndValues ...interface{})
|
|
}
|
|
|
|
type pLogger struct {
|
|
prefix string
|
|
depth int
|
|
}
|
|
|
|
func New(prefix string) Logger {
|
|
return &pLogger{
|
|
depth: 0,
|
|
prefix: prefix,
|
|
}
|
|
}
|
|
|
|
func (p *pLogger) Error(msg string, err error, keysAndValues ...interface{}) {
|
|
klog.ErrorSDepth(p.depth+1, err, p.prefix+msg, keysAndValues...)
|
|
}
|
|
|
|
func (p *pLogger) warningDepth(msg string, depth int, keysAndValues ...interface{}) {
|
|
// klog's structured logging has no concept of a warning (i.e. no WarningS function)
|
|
// Thus we use info at log level zero as a proxy
|
|
// klog's info logs have an I prefix and its warning logs have a W prefix
|
|
// Since we lose the W prefix by using InfoS, just add a key to make these easier to find
|
|
keysAndValues = append([]interface{}{"warning", "true"}, keysAndValues...)
|
|
if klog.V(klogLevelWarning).Enabled() {
|
|
klog.InfoSDepth(depth+1, p.prefix+msg, keysAndValues...)
|
|
}
|
|
}
|
|
|
|
func (p *pLogger) Warning(msg string, keysAndValues ...interface{}) {
|
|
p.warningDepth(msg, p.depth+1, keysAndValues...)
|
|
}
|
|
|
|
// Use WarningErr to issue a Warning message with an error object as part of the message.
|
|
func (p *pLogger) WarningErr(msg string, err error, keysAndValues ...interface{}) {
|
|
p.warningDepth(msg, p.depth+1, append([]interface{}{errorKey, err}, keysAndValues...)...)
|
|
}
|
|
|
|
func (p *pLogger) infoDepth(msg string, depth int, keysAndValues ...interface{}) {
|
|
if klog.V(klogLevelInfo).Enabled() {
|
|
klog.InfoSDepth(depth+1, p.prefix+msg, keysAndValues...)
|
|
}
|
|
}
|
|
|
|
func (p *pLogger) Info(msg string, keysAndValues ...interface{}) {
|
|
p.infoDepth(msg, p.depth+1, keysAndValues...)
|
|
}
|
|
|
|
// Use InfoErr to log an expected error, e.g. validation failure of an http parameter.
|
|
func (p *pLogger) InfoErr(msg string, err error, keysAndValues ...interface{}) {
|
|
p.infoDepth(msg, p.depth+1, append([]interface{}{errorKey, err}, keysAndValues...)...)
|
|
}
|
|
|
|
func (p *pLogger) debugDepth(msg string, depth int, keysAndValues ...interface{}) {
|
|
if klog.V(klogLevelDebug).Enabled() {
|
|
klog.InfoSDepth(depth+1, p.prefix+msg, keysAndValues...)
|
|
}
|
|
}
|
|
|
|
func (p *pLogger) Debug(msg string, keysAndValues ...interface{}) {
|
|
p.debugDepth(msg, p.depth+1, keysAndValues...)
|
|
}
|
|
|
|
// Use DebugErr to issue a Debug message with an error object as part of the message.
|
|
func (p *pLogger) DebugErr(msg string, err error, keysAndValues ...interface{}) {
|
|
p.debugDepth(msg, p.depth+1, append([]interface{}{errorKey, err}, keysAndValues...)...)
|
|
}
|
|
|
|
func (p *pLogger) traceDepth(msg string, depth int, keysAndValues ...interface{}) {
|
|
if klog.V(klogLevelTrace).Enabled() {
|
|
klog.InfoSDepth(depth+1, p.prefix+msg, keysAndValues...)
|
|
}
|
|
}
|
|
|
|
func (p *pLogger) Trace(msg string, keysAndValues ...interface{}) {
|
|
p.traceDepth(msg, p.depth+1, keysAndValues...)
|
|
}
|
|
|
|
// Use TraceErr to issue a Trace message with an error object as part of the message.
|
|
func (p *pLogger) TraceErr(msg string, err error, keysAndValues ...interface{}) {
|
|
p.traceDepth(msg, p.depth+1, append([]interface{}{errorKey, err}, keysAndValues...)...)
|
|
}
|
|
|
|
func (p *pLogger) All(msg string, keysAndValues ...interface{}) {
|
|
if klog.V(klogLevelAll).Enabled() {
|
|
klog.InfoSDepth(p.depth+1, p.prefix+msg, keysAndValues...)
|
|
}
|
|
}
|
|
|
|
var logger Logger = &pLogger{ //nolint:gochecknoglobals
|
|
depth: 1,
|
|
}
|
|
|
|
// Use Error to log an unexpected system error.
|
|
func Error(msg string, err error, keysAndValues ...interface{}) {
|
|
logger.Error(msg, err, keysAndValues...)
|
|
}
|
|
|
|
func Warning(msg string, keysAndValues ...interface{}) {
|
|
logger.Warning(msg, keysAndValues...)
|
|
}
|
|
|
|
// Use WarningErr to issue a Warning message with an error object as part of the message.
|
|
func WarningErr(msg string, err error, keysAndValues ...interface{}) {
|
|
logger.WarningErr(msg, err, keysAndValues...)
|
|
}
|
|
|
|
func Info(msg string, keysAndValues ...interface{}) {
|
|
logger.Info(msg, keysAndValues...)
|
|
}
|
|
|
|
// Use InfoErr to log an expected error, e.g. validation failure of an http parameter.
|
|
func InfoErr(msg string, err error, keysAndValues ...interface{}) {
|
|
logger.InfoErr(msg, err, keysAndValues...)
|
|
}
|
|
|
|
func Debug(msg string, keysAndValues ...interface{}) {
|
|
logger.Debug(msg, keysAndValues...)
|
|
}
|
|
|
|
// Use DebugErr to issue a Debug message with an error object as part of the message.
|
|
func DebugErr(msg string, err error, keysAndValues ...interface{}) {
|
|
logger.DebugErr(msg, err, keysAndValues...)
|
|
}
|
|
|
|
func Trace(msg string, keysAndValues ...interface{}) {
|
|
logger.Trace(msg, keysAndValues...)
|
|
}
|
|
|
|
// Use TraceErr to issue a Trace message with an error object as part of the message.
|
|
func TraceErr(msg string, err error, keysAndValues ...interface{}) {
|
|
logger.TraceErr(msg, err, keysAndValues...)
|
|
}
|
|
|
|
func All(msg string, keysAndValues ...interface{}) {
|
|
logger.All(msg, keysAndValues...)
|
|
}
|