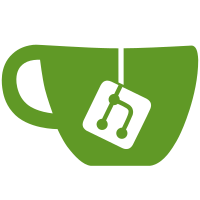
- For backwards compatibility with older Pinniped CLIs, the pinniped-cli client does not need to request the username or groups scopes for them to be granted. For dynamic clients, the usual OAuth2 rules apply: the client must be allowed to request the scopes according to its configuration, and the client must actually request the scopes in the authorization request. - If the username scope was not granted, then there will be no username in the ID token, and the cluster-scoped token exchange will fail since there would be no username in the resulting cluster-scoped ID token. - The OIDC well-known discovery endpoint lists the username and groups scopes in the scopes_supported list, and lists the username and groups claims in the claims_supported list. - Add username and groups scopes to the default list of scopes put into kubeconfig files by "pinniped get kubeconfig" CLI command, and the default list of scopes used by "pinniped login oidc" when no list of scopes is specified in the kubeconfig file - The warning header about group memberships changing during upstream refresh will only be sent to the pinniped-cli client, since it is only intended for kubectl and it could leak the username to the client (which may not have the username scope granted) through the warning message text. - Add the user's username to the session storage as a new field, so that during upstream refresh we can compare the original username from the initial authorization to the refreshed username, even in the case when the username scope was not granted (and therefore the username is not stored in the ID token claims of the session storage) - Bump the Supervisor session storage format version from 2 to 3 due to the username field being added to the session struct - Extract commonly used string constants related to OIDC flows to api package. - Change some import names to make them consistent: - Always import github.com/coreos/go-oidc/v3/oidc as "coreosoidc" - Always import go.pinniped.dev/generated/latest/apis/supervisor/oidc as "oidcapi" - Always import go.pinniped.dev/internal/oidc as "oidc"
59 lines
1.7 KiB
Go
59 lines
1.7 KiB
Go
// Copyright 2020-2022 the Pinniped contributors. All Rights Reserved.
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
// Package nonce implements helpers for OIDC nonce parameter handling.
|
|
package nonce
|
|
|
|
import (
|
|
"crypto/rand"
|
|
"crypto/subtle"
|
|
"encoding/hex"
|
|
"fmt"
|
|
"io"
|
|
|
|
coreosoidc "github.com/coreos/go-oidc/v3/oidc"
|
|
"golang.org/x/oauth2"
|
|
)
|
|
|
|
// Generate generates a new random OIDC nonce parameter of an appropriate size.
|
|
func Generate() (Nonce, error) { return generate(rand.Reader) }
|
|
|
|
func generate(rand io.Reader) (Nonce, error) {
|
|
var buf [16]byte
|
|
if _, err := io.ReadFull(rand, buf[:]); err != nil {
|
|
return "", fmt.Errorf("could not generate random nonce: %w", err)
|
|
}
|
|
return Nonce(hex.EncodeToString(buf[:])), nil
|
|
}
|
|
|
|
// Nonce implements some utilities for working with OIDC nonce parameters.
|
|
type Nonce string
|
|
|
|
// String returns the string encoding of this state value.
|
|
func (n *Nonce) String() string {
|
|
return string(*n)
|
|
}
|
|
|
|
// Param returns the OAuth2 auth code parameter for sending the nonce during the authorization request.
|
|
func (n *Nonce) Param() oauth2.AuthCodeOption {
|
|
return coreosoidc.Nonce(string(*n))
|
|
}
|
|
|
|
// Validate the returned ID token). Returns true iff the nonce matches or the returned JWT does not have a nonce.
|
|
func (n *Nonce) Validate(token *coreosoidc.IDToken) error {
|
|
if subtle.ConstantTimeCompare([]byte(token.Nonce), []byte(*n)) != 1 {
|
|
return InvalidNonceError{Expected: *n, Got: Nonce(token.Nonce)}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// InvalidNonceError is returned by Validate when the observed nonce is invalid.
|
|
type InvalidNonceError struct {
|
|
Expected Nonce
|
|
Got Nonce
|
|
}
|
|
|
|
func (e InvalidNonceError) Error() string {
|
|
return fmt.Sprintf("invalid nonce (expected %q, got %q)", e.Expected.String(), e.Got.String())
|
|
}
|