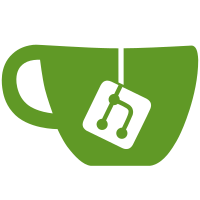
- Add `AllowPasswordGrant` boolean field to OIDCIdentityProvider's spec - The oidc upstream watcher controller copies the value of `AllowPasswordGrant` into the configuration of the cached provider - Add password grant to the UpstreamOIDCIdentityProviderI interface which is implemented by the cached provider instance for use in the authorization endpoint - Enhance the IDP discovery endpoint to return the supported "flows" for each IDP ("cli_password" and/or "browser_authcode") - Enhance `pinniped get kubeconfig` to help the user choose the desired flow for the selected IDP, and to write the flow into the resulting kubeconfg - Enhance `pinniped login oidc` to have a flow flag to tell it which client-side flow it should use for auth (CLI-based or browser-based) - In the Dex config, allow the resource owner password grant, which Dex implements to also return ID tokens, for use in integration tests - Enhance the authorize endpoint to perform password grant when requested by the incoming headers. This commit does not include unit tests for the enhancements to the authorize endpoint, which will come in the next commit - Extract some shared helpers from the callback endpoint to share the code with the authorize endpoint - Add new integration tests
188 lines
7.7 KiB
Go
188 lines
7.7 KiB
Go
// Copyright 2020-2021 the Pinniped contributors. All Rights Reserved.
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
//
|
|
|
|
// Code generated by MockGen. DO NOT EDIT.
|
|
// Source: go.pinniped.dev/internal/oidc/provider (interfaces: UpstreamOIDCIdentityProviderI)
|
|
|
|
// Package mockupstreamoidcidentityprovider is a generated GoMock package.
|
|
package mockupstreamoidcidentityprovider
|
|
|
|
import (
|
|
context "context"
|
|
url "net/url"
|
|
reflect "reflect"
|
|
|
|
gomock "github.com/golang/mock/gomock"
|
|
nonce "go.pinniped.dev/pkg/oidcclient/nonce"
|
|
oidctypes "go.pinniped.dev/pkg/oidcclient/oidctypes"
|
|
pkce "go.pinniped.dev/pkg/oidcclient/pkce"
|
|
oauth2 "golang.org/x/oauth2"
|
|
)
|
|
|
|
// MockUpstreamOIDCIdentityProviderI is a mock of UpstreamOIDCIdentityProviderI interface.
|
|
type MockUpstreamOIDCIdentityProviderI struct {
|
|
ctrl *gomock.Controller
|
|
recorder *MockUpstreamOIDCIdentityProviderIMockRecorder
|
|
}
|
|
|
|
// MockUpstreamOIDCIdentityProviderIMockRecorder is the mock recorder for MockUpstreamOIDCIdentityProviderI.
|
|
type MockUpstreamOIDCIdentityProviderIMockRecorder struct {
|
|
mock *MockUpstreamOIDCIdentityProviderI
|
|
}
|
|
|
|
// NewMockUpstreamOIDCIdentityProviderI creates a new mock instance.
|
|
func NewMockUpstreamOIDCIdentityProviderI(ctrl *gomock.Controller) *MockUpstreamOIDCIdentityProviderI {
|
|
mock := &MockUpstreamOIDCIdentityProviderI{ctrl: ctrl}
|
|
mock.recorder = &MockUpstreamOIDCIdentityProviderIMockRecorder{mock}
|
|
return mock
|
|
}
|
|
|
|
// EXPECT returns an object that allows the caller to indicate expected use.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) EXPECT() *MockUpstreamOIDCIdentityProviderIMockRecorder {
|
|
return m.recorder
|
|
}
|
|
|
|
// AllowsPasswordGrant mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) AllowsPasswordGrant() bool {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "AllowsPasswordGrant")
|
|
ret0, _ := ret[0].(bool)
|
|
return ret0
|
|
}
|
|
|
|
// AllowsPasswordGrant indicates an expected call of AllowsPasswordGrant.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) AllowsPasswordGrant() *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "AllowsPasswordGrant", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).AllowsPasswordGrant))
|
|
}
|
|
|
|
// ExchangeAuthcodeAndValidateTokens mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) ExchangeAuthcodeAndValidateTokens(arg0 context.Context, arg1 string, arg2 pkce.Code, arg3 nonce.Nonce, arg4 string) (*oidctypes.Token, error) {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "ExchangeAuthcodeAndValidateTokens", arg0, arg1, arg2, arg3, arg4)
|
|
ret0, _ := ret[0].(*oidctypes.Token)
|
|
ret1, _ := ret[1].(error)
|
|
return ret0, ret1
|
|
}
|
|
|
|
// ExchangeAuthcodeAndValidateTokens indicates an expected call of ExchangeAuthcodeAndValidateTokens.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) ExchangeAuthcodeAndValidateTokens(arg0, arg1, arg2, arg3, arg4 interface{}) *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "ExchangeAuthcodeAndValidateTokens", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).ExchangeAuthcodeAndValidateTokens), arg0, arg1, arg2, arg3, arg4)
|
|
}
|
|
|
|
// GetAuthorizationURL mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) GetAuthorizationURL() *url.URL {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "GetAuthorizationURL")
|
|
ret0, _ := ret[0].(*url.URL)
|
|
return ret0
|
|
}
|
|
|
|
// GetAuthorizationURL indicates an expected call of GetAuthorizationURL.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) GetAuthorizationURL() *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "GetAuthorizationURL", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).GetAuthorizationURL))
|
|
}
|
|
|
|
// GetClientID mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) GetClientID() string {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "GetClientID")
|
|
ret0, _ := ret[0].(string)
|
|
return ret0
|
|
}
|
|
|
|
// GetClientID indicates an expected call of GetClientID.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) GetClientID() *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "GetClientID", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).GetClientID))
|
|
}
|
|
|
|
// GetGroupsClaim mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) GetGroupsClaim() string {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "GetGroupsClaim")
|
|
ret0, _ := ret[0].(string)
|
|
return ret0
|
|
}
|
|
|
|
// GetGroupsClaim indicates an expected call of GetGroupsClaim.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) GetGroupsClaim() *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "GetGroupsClaim", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).GetGroupsClaim))
|
|
}
|
|
|
|
// GetName mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) GetName() string {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "GetName")
|
|
ret0, _ := ret[0].(string)
|
|
return ret0
|
|
}
|
|
|
|
// GetName indicates an expected call of GetName.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) GetName() *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "GetName", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).GetName))
|
|
}
|
|
|
|
// GetScopes mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) GetScopes() []string {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "GetScopes")
|
|
ret0, _ := ret[0].([]string)
|
|
return ret0
|
|
}
|
|
|
|
// GetScopes indicates an expected call of GetScopes.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) GetScopes() *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "GetScopes", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).GetScopes))
|
|
}
|
|
|
|
// GetUsernameClaim mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) GetUsernameClaim() string {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "GetUsernameClaim")
|
|
ret0, _ := ret[0].(string)
|
|
return ret0
|
|
}
|
|
|
|
// GetUsernameClaim indicates an expected call of GetUsernameClaim.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) GetUsernameClaim() *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "GetUsernameClaim", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).GetUsernameClaim))
|
|
}
|
|
|
|
// PasswordCredentialsGrantAndValidateTokens mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) PasswordCredentialsGrantAndValidateTokens(arg0 context.Context, arg1, arg2 string) (*oidctypes.Token, error) {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "PasswordCredentialsGrantAndValidateTokens", arg0, arg1, arg2)
|
|
ret0, _ := ret[0].(*oidctypes.Token)
|
|
ret1, _ := ret[1].(error)
|
|
return ret0, ret1
|
|
}
|
|
|
|
// PasswordCredentialsGrantAndValidateTokens indicates an expected call of PasswordCredentialsGrantAndValidateTokens.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) PasswordCredentialsGrantAndValidateTokens(arg0, arg1, arg2 interface{}) *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "PasswordCredentialsGrantAndValidateTokens", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).PasswordCredentialsGrantAndValidateTokens), arg0, arg1, arg2)
|
|
}
|
|
|
|
// ValidateToken mocks base method.
|
|
func (m *MockUpstreamOIDCIdentityProviderI) ValidateToken(arg0 context.Context, arg1 *oauth2.Token, arg2 nonce.Nonce) (*oidctypes.Token, error) {
|
|
m.ctrl.T.Helper()
|
|
ret := m.ctrl.Call(m, "ValidateToken", arg0, arg1, arg2)
|
|
ret0, _ := ret[0].(*oidctypes.Token)
|
|
ret1, _ := ret[1].(error)
|
|
return ret0, ret1
|
|
}
|
|
|
|
// ValidateToken indicates an expected call of ValidateToken.
|
|
func (mr *MockUpstreamOIDCIdentityProviderIMockRecorder) ValidateToken(arg0, arg1, arg2 interface{}) *gomock.Call {
|
|
mr.mock.ctrl.T.Helper()
|
|
return mr.mock.ctrl.RecordCallWithMethodType(mr.mock, "ValidateToken", reflect.TypeOf((*MockUpstreamOIDCIdentityProviderI)(nil).ValidateToken), arg0, arg1, arg2)
|
|
}
|